The DynaPDF version 4.0.62.160 showed up and features the use of the new \dl# tag to double underline text.
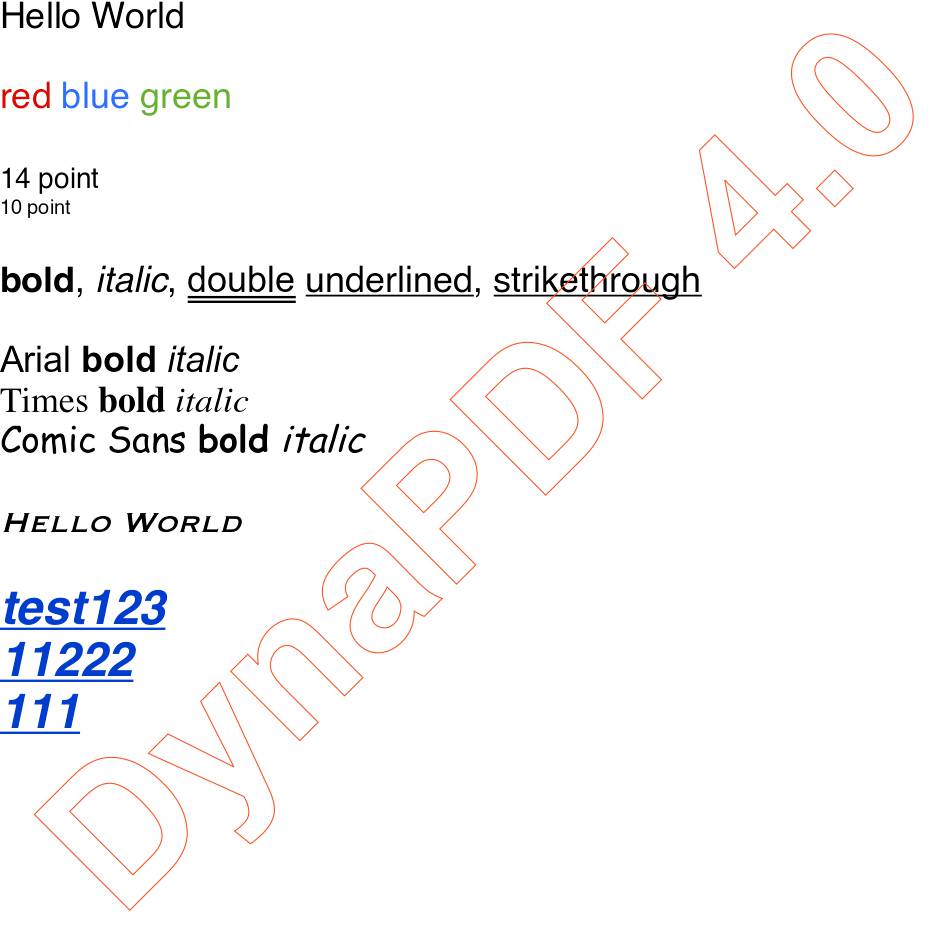
Our code for
DynaPDF.ConvertStyledText in the
MBS FileMaker Plugin got updated and other functions like
DynaPDF.WriteStyledText will use this, too. So you can now pass styled text from FileMaker to our plugin and we can convert the styled text and preserve the double underline.
Coming soon with next pre-release.
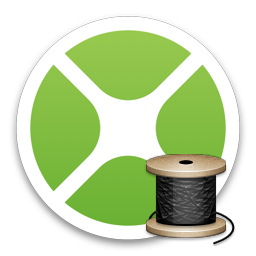
For our
MBS Xojo Plugins we add a thread pooling for Windows in version 20.4. Instead of having the plugin create threads if needed for doing work and reuse them if needed.
For version 20.5 we got a new class to control that:
WinThreadPoolMBS
We go these four properties:
- StackCommit as Integer
- StackReserve as Integer
- ThreadMaximum as Integer
- ThreadMinimum as Integer
The default stack size is 1 MB with a grow rate of 4 KB. Thread minimum and maximum is not limited, so Windows can decide based on CPU core count and load.
If you don't use the class, you get the default behavior: a shared thread pool for the whole process. This may also be used by plugins from other vendors.
But if you use this class, we create a thread pool for our MBS Plugin functions and you can configure how much we use it. In case you use this, please initialize this early and keep the object around, e.g. put in a property of app or a module.
Please try the current pre-release version of the plugin to see how it works for you.

For many years we used AddressBook framework to interact with the built-in AddressBook application on macOS. Since about macOS 10.2 from 2002 those classes have been in the operation system. Since OS X El Capitan 10.11 from 2015 we got the
Contacts framework to replace the older AddressBook classes and provide access to the same database for contacts on your application. Somewhere in-between macOS release, the applications for end users got rewritten and renamed to Contacts.app.
The AddressBook plugin is deprecated for a few years and it looks like finally Apple put it on the no-go list for the App Store. At least we got such a notice for the ABPersonView control in one fo the applications of a client. This is one of the controls we provide for address book access.
We already marked AddressBook functions as deprecated, but it may be finally time for you to look through your code and remove all references to AB* classes:
class
ABAccountMBS
class
ABAddressBookMBS
class
ABGroupMBS
class
ABMultiValueMBS
class
ABMutableMultiValueMBS
class
ABPersonMBS
class
ABPickerMBS
class
ABRecordMBS
class
ABSearchElementMBS
class
ABPeoplePickerViewMBS
class
ABPersonViewMBS
control
ABPeoplePickerViewControlMBS
control
ABPersonViewControlMBS
Let us know if you have questions on the transition to
Contacts framework or if something is missing in our plugins.
For
MBS Xojo Plugins we plan to remove AddressBook completely in a cleanup in winter, so 2022 versions may no longer have it. Because if Apple starts to flag those classes as no longer being eligible for App Store submission, it's time to say goodbye.
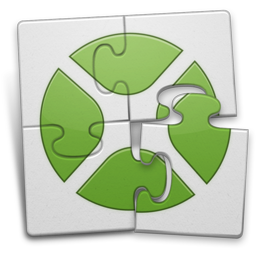
New in this prerelease of the 21.5 plugins:
Download:
monkeybreadsoftware.com/xojo/download/plugin/Prerelease/ or
from Dropbox.
Or ask us to be added to our shared Dropbox folder.
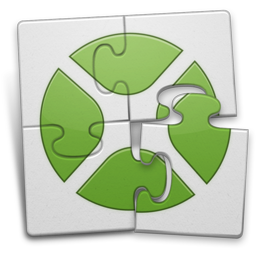
New in this prerelease of the 21.5 plugins:
Download:
monkeybreadsoftware.com/xojo/download/plugin/Prerelease/ or
from Dropbox.
Or ask us to be added to our shared Dropbox folder.
If you like to get styled text on your labels in iOS, you can use our NSAttributedStringMBS class in our MBS Xojo MacCocoa Plugin like below.
As you see we create a NSMutableAttributedStringMBS object for some string and add attributes with zero based ranges there. We then use a declare to setAttributedText to assign the attributed string. Currently our classes for Cocoa controls are not ported to iOS in the plugin, but a quick declare helps us here.
EventHandler Sub Open()
// create some text from a string. Could also read in RTF
Dim a As NSAttributedStringMBS = NSAttributedStringMBS.attributedStringWithString("Hello World")
// make a mutable copy
Dim m As NSMutableAttributedStringMBS = a.mutableCopy
// now add bold font
Dim r1 As New NSRangeMBS(0,5)
m.addAttribute(m.NSFontAttributeName, NSFontMBS.boldSystemFontOfSize(13), r1)
// and underline
Dim r2 As New NSRangeMBS(6,5)
m.addAttribute(m.NSUnderlineStyleAttributeName, m.NSUnderlineStyleSingle, r2)
// assign new styled text
Declare Sub setAttributedText Lib "UIKit" Selector "setAttributedText:" (NSTextViewRef As ptr, AttributedStringHandle As Integer )
setAttributedText Label1.Handle, m.Handle
End EventHandler
Not all styled are available on iOS. You can for example use NSForegroundColorAttributeName with NSColorMBS (actually UIColor on iOS internally) to color the text. Since superscript and subscript are not available, you can emulate it with using NSBaselineOffsetAttributeName to change the base line and then use a smaller font for the words.
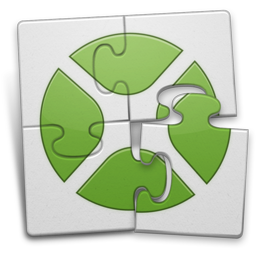
New in this prerelease of the 21.5 plugins:
- Fixed code to return FolderItem in various functions on iOS.
- Updated DynaPDF to version 4.0.59.157.
- Changed MLFeatureValueMBS.featureValueWithPicture to convert picture to bitmap if needed.
- Enabled ConsoleExecuteMBS method for desktop projects.
- Fixed issue with CloudKit classes, where CKShareMBS was returned CKRecordMBS.
- Added CacheInsertStatement flag for SQLDatabaseMBS to cache insert statements for InsertRecord to speed those up.
- Added StartIndex parameter to FindValueInArray and FindValueInObjectArray methods in JSONMBS class, so you can repeat search until nothing more is found.
- Added new constructor for DirectShowEnumMonikerMBS to find all filters available.
- Added new events for NSSharingServiceDelegateMBS class for cloud sharing.
Download:
monkeybreadsoftware.com/xojo/download/plugin/Prerelease/ or
from Dropbox.
Or ask us to be added to our shared Dropbox folder.
We got a new example for our MBS Xojo DynaPDF Plugin for using DynaPDFMBS class to create a gradient with DeviceN color space. We use DynaPDFExtGStateMBS to set the extended graphics state and enable overprint mode. Then we create the DevcieN colorspace based on a CMYK fallback. And within that colorspace we define an axial shading to get a gradient to fill an area in the PDF page. Here is the new sample code:
// Draw something in background so that we can see whether it works
Call pdf.SetColorSpace(pdf.kcsDeviceCMYK)
Call pdf.SetFillColor(PDF.CMYK(0, 0, 255, 0))
Call pdf.Rectangle(0.0, 0.0, 595.0, 842.0, pdf.kfmFill)
Dim gs As New DynaPDFExtGStateMBS
gs.OverPrintMode = 1
gs.OverPrintFill = 1
Dim gsHandle As Integer = pdf.CreateExtGState(gs)
Call pdf.SetExtGState(gsHandle)
// In this example, we emulate a CMYK color space with a DeviceN color space.
// The required Postscript calculator function is super simple, it consists of only two braces"{}".
Dim cls() As String = Array("Cyan", "Magenta", "Yellow", "Black")
Dim cs As Integer = pdf.CreateDeviceNColorSpace(cls, "{}", pdf.kesDeviceCMYK, -1)
Call pdf.SetExtFillColorSpace(cs)
Dim sh As Integer = pdf.CreateAxialShading( 50.0, 50.0, 550.0, 50.0, 0.5, PDF.CMYK(60, 0, 0, 0), PDF.CMYK(255, 0, 0, 0), False, False)
Call pdf.ApplyShading(sh)
This needs a DynaPDF Pro license from us for the plugin. Feel free to try the example project included with future MBS Plugin releases and send us questions.
Due to current events in an office nearby, here again an announcement announcement for all database developers:
Please treat the following numbers as text for processing and storing in a database.
Zip codes
While we call them zip numbers in Germany and they are just five digits here, a zip code may contain various other characters like letters, spaces and dashes. e.g. "SW1A 1AA" is a valid zip code in the United Kingdom. The length of post codes may be over 10 characters in some places and contain leading zeros. In Argentina even a plus may be used like "46C2+32".
Invoice Numbers
The number on an invoice, an offer or an order often has a scheme defined by the used software. Some companies use dashes to separate sections of the number like year-branch-number to give each shop branch their own running count for invoices. We have seen invoices numbers with prefix or postfix to indicate branches or types of invoices.
(more)
When to initialize what is always a good question. Doing a custom constructor for Application, a Window or Control allows you to initialize some things before Xojo does some setup steps. For example if you like to have custom delegates for Cocoa frameworks, like our
NSApplicationDelegateMBS class. The MBS Plugin registration can also either go into the constructor or App.Open event.
The constructor of a control allows you to do initialization steps before the control is added to the window. The open event of the control then allows you to do things after the control is initialized. And the open event of the window allows you to setup something after all controls are setup, e.g. connect a window splitter to the left and right controls.
(more)
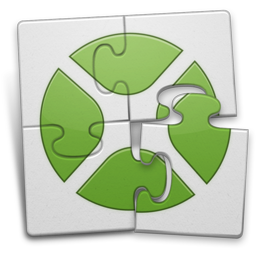
New in this prerelease of the 21.5 plugins:
- Added AddSort method to XLAutoFilterMBS class.
- Added DynaPDFVersionInfoMBS class.
- Added ErrCode and ErrType properties to DynaPDFErrorMBS class.
- Added GetPDFVersionEx function for DynaPDFMBS class.
- Added IsAutoFilter function to XLSheetMBS class.
- Added ScreenResolution property to DynaPDFRasterizerMBS class.
- Added XLSFormControlMBS class and related methods.
- Changed a few old deprecated controls to hide in the control library.
- Changed DynaPDF examples to use kcpUnicode instead of kcp1252.
- Changed PermissionsMBS class to be marked as console safe.
- Changed SQLPreparedStatementMBS class to raise TypeMismatchException if you pass array of variant for param array, e.g. array in array.
- Deprecated LoadString in ChromiumFrameMBS class since CEF deprecated the method.
- Fixed memory leak for ZintVectorStringMBS and ZintVectorStringMBS classes.
- Fixed memory leak in BarcodeGeneratorMBS class.
- Fixed NSSearchFieldControlMBS class to fire KeyDown/KeyUp events for return/enter keys, too.
- Fixed passing of numbers for SetStrokeColorEx() taking param array.
- Fixed socket methods for PacketSocketMBS class.
- Updated Chromium classes to newer CEF version.
- Updated CURL library to version 7.79.1.
- Updated DynaPDF to version 4.0.59.156.
- Updated LibXL to version 4.0.
- Updated SQLAPI to version 5.1.6.
Download:
monkeybreadsoftware.com/xojo/download/plugin/Prerelease/ or
from Dropbox.
Or ask us to be added to our shared Dropbox folder.